If you are a React developer, you may have already run into React useMemo vs useCallback discussions online and ended up more confused than ever before. It can be very hard figuring out the difference between useMemo and useCallback. That’s OK! It can be difficult to get your head around React-Hooks in the beginning, but they can be extremely useful down the line.
In this article, we’ll show you the difference between useCallback and useMemo and when to use useCallback and useMemo.
The Difference Between useMemo and useCallback
Two built-in hooks were introduced with React 16.8. These features were introduced to optimize the performance. The difference between useMemo vs useCallback seems negligible, so we’ll try to clean up the confusion.
What are React-Hooks?
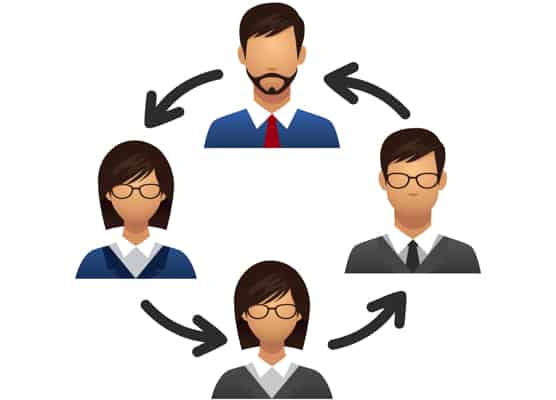
Hooks are new features introduced in React that allow the useState and other React features without writing a class. Hooks are methods and functions that “hook” onto React’s state and lifecycle features.
useCallback vs useMemo
The useCallback hook is used whenever a child component is re-rendering over and over again without really needing to. When you use useCallback, you can prevent this unnecessary re-rendering by returning the same instance of that function that is being passed instead of creating a new instance every single time. This can save a considerable amount of time (and money spent on dev hours). Passing an array of dependencies with a memoized callback will result in a memoized version that will only be changed when the dependence changes.
useMemo is used in the functional component of React. It’s used to return a memoized value. Memoization refers to the process of saving re-compilation time by returning a cached result instead of recompiling a function with the same argument again for the next run. The cached result is returned the next time it is called. useMemo hooks provide memory-mapped values.
Clearly, there are some similarities. In both useMemo and useCallback, the hook accepts a function as well as an array of dependencies. However, useCallback will remember the returned value, and useMemo will remember the function. In other words, one caches a value type, and the other caches a function.
- READ MORE – How and Why You Should Keep Your Files Organized
- Top 9 Ways To Make Use Of Electronic Gadgets In Classroom
When to use useCallback vs useMemo
So when should you use useCallback, and when is useMemo the best option? Let’s look at an example. If a computationally heavy and expensive code accepts arguments and returns a value, useMemo is the best option. That way, you can keep referencing the value between the renders without re-running the code and wasting your time and computational power.
If you need to keep a function instance persistent across multiple renders, you should use useCallback instead because it essentially places the function outside the scope of your React component to keep it intact.
What is useEffect?
We’ve already made mention of the dependency array, which you may have encountered when you use useEffect. useEffect is another React hook. It’s used to handle side-effects in functional components using the callback argument logic. Dependencies are the list of dependencies of the side-effect, e.g., props or state values.
This hook shows React that the component needs to perform an action after rendering. When the DOM is updated, the effect you pass will be stored. You can also perform data fetching to achieve this result.
In short, useCallback, useMemo, and useEffect are all concerned with enhancing the performance between re-rendering of components in React applications.
It’s important not to overuse hooks, though. The hooks introduce new and complex logic, and it could potentially have the opposite effect than you intended, creating performance issues instead of solving them. useMemo should only be used for really expensive computations.
Don’t use useMemo or useCallback to fix fundamental issues with your application. Eventually, these issues will resurface and haunt you, so best attend to any known faults first.
Conclusion
Hopefully, this article was insightful, and you understand the two hooks better. In summary:
- Both accept a function and array of dependencies
- useMemo memorizes the value returned, useCallback memorizes the function.
The difference between useMemo and useCallback is pretty clear! These tools have the potential of saving time and money, but only if they are used in the proper context and environment.
I hope this tutorial helped you to know about the When To Use useMemo and useCallback: a Brief Guide For React Fans. If you want to say anything, let us know through the comment sections. If you like this article, please share it and follow WhatVwant on Facebook, Twitter, and YouTube for more Technical tips.
When To Use useMemo and useCallback – FAQs
What is the difference between useCallback and useMemo?
UseCallsback is used to optimize the rendering behavior of your React function components, while useMemo is used to memorize expensive functions to avoid having to call them on every render.
When should useCallback be used?
The useCallback hook is used when you have a component in which the child is rendering again and again without need.
Why do we use useMemo?
useMemo is one of the hooks offered by React. This hook allows developers to cache the value of a variable along with a dependency list.
Does useMemo trigger re-render?
Yes, that is entirely possible. What useMemo does is after a rerender occurs, it compares the values in its dependency array, if they didn’t change then the last computed value is returned, otherwise it recomputes.
How do I use useEffect and useCallback?
The only way useEffect code can do anything visible is by either changing the state to cause a re-render or modifying the DOM directly.
Is useMemo shallow?
memo uses a shallow comparison of the component props and because of how JavaScript works, comparing objects shallowly will return false even if they have the same values.